Reactのお勉強つづき。
以前にVue.jsのお勉強時に作成したTODOリストをReactで同じように作ってみます。
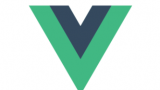
Vue.jsでToDoList
Vue.jsのお勉強。とりあえずデータの保存は置いておいて、・TODOリストの追加、削除・チェックボックスにチェックで取り消し線表示を実装するまで。操作イメージは以下のような感じで。HTML<html lang="jp"><head> <l...
create-react-appで作成された App.js と App.css を編集して、出来上がったソースは以下になります。
App.js
import React, { Component } from 'react'; import './App.css'; class App extends Component { constructor(props){ super(props); this.state = { todos: [{item: "test", isDone: false}] }; this.additem = this.additem.bind(this); } // item追加 additem(){ // 入力が空だったら何もしない if(this.refs.newItem.value === '') return; var todo = { item: this.refs.newItem.value, isDone: false, }; this.state.todos.push(todo); this.setState(this.state.todos); this.refs.newItem.value=""; } // item削除 delitem(index){ this.state.todos.splice(index, 1); this.setState(this.state.todos); } // item完了・未完了の切り替え doneitem(index){ var todo = this.state.todos[index]; todo.isDone = todo.isDone ? false : true this.setState(this.state.todos); } render() { return ( <div> <h2>TODO List</h2> <input type="text" ref="newItem"/> <button onClick={this.additem}> Add </button> <ul> {this.state.todos.map((todo, index) => { if(todo.isDone){ // 完了済みの場合、取り消し線を付ける<span>タグを出す return <li key={index}><input type="checkbox" onClick={() => this.doneitem(index)}/><span className="done">{todo.item}</span><input type="button" value="delete" onClick={() => this.delitem(index)}/></li> }else{ return <li key={index}><input type="checkbox" onClick={() => this.doneitem(index)} />{todo.item}<input type="button" value="delete" onClick={() => this.delitem(index)}/></li> } })} </ul> </div> ); } } export default App;
App.css
ul{ list-style: none; } li > span.done { text-decoration: line-through; }
このくらいのお試し動作だと、Vue.jsの方がとっつきやすい印象です。
コメント